reetry capsule(Retrying Failed API Requests with Retry Capsule in Python)
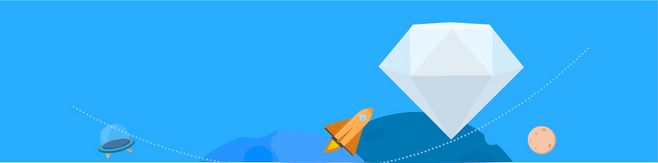
Retrying Failed API Requests with Retry Capsule in Python
Introduction
API requests are quite common for developers while building applications. Sometimes, due to network issues, the API requests may fail. One way to handle this issue is by retrying the request after a certain interval. In this article, we will discuss how to use the retry capsule library in Python to retry failed API requests.
Getting Started with Retry Capsule
First, let's install the retry capsule library using pip:
pip install retry-capsule
Once installed, we can import the retry capsule library into our Python script:
import retry_capsule
Retrying API Requests
Let's say we have an API endpoint that we want to call and get data from. We can use the requests module in Python to make HTTP requests to the API. Here's an example:
import requestsdef call_api(): try: response = requests.get(\"https://api.example.com/data\") response.raise_for_status() # Check if the response is successful except requests.exceptions.HTTPError as err: raise SystemExit(err) return response.json()
In the example above, we are using the requests module to make a GET request to https://api.example.com/data. We then check if the response is successful using the raise_for_status() method. If the response is not successful, we raise an HTTPError exception.
Now, let's say we want to retry this API call if it fails due to a network issue. We can use the retry_capsule library to do this. Here's an example:
import requestsimport retry_capsule@retry_capsule.retry(max_attempts=3, delay=1)def call_api(): try: response = requests.get(\"https://api.example.com/data\") response.raise_for_status() # Check if the response is successful except requests.exceptions.HTTPError as err: raise retry_capsule.RetryError(err) return response.json()
In the example above, we are using the retry_capsule.retry() decorator to retry the call_api() function up to 3 times with a delay of 1 second between each attempt. If the API call fails, we catch the HTTPError exception and raise a RetryError exception, which tells the retry capsule library to retry the function call.
Conclusion
In this article, we learned how to use the retry capsule library in Python to retry failed API requests. By using this library, we can handle network issues and retry failed API requests automatically, without the need for manual intervention.
Try using the retry capsule library in your next project where you need to make API requests and see the benefits it can bring.